ReactJS 18 guide for beginners
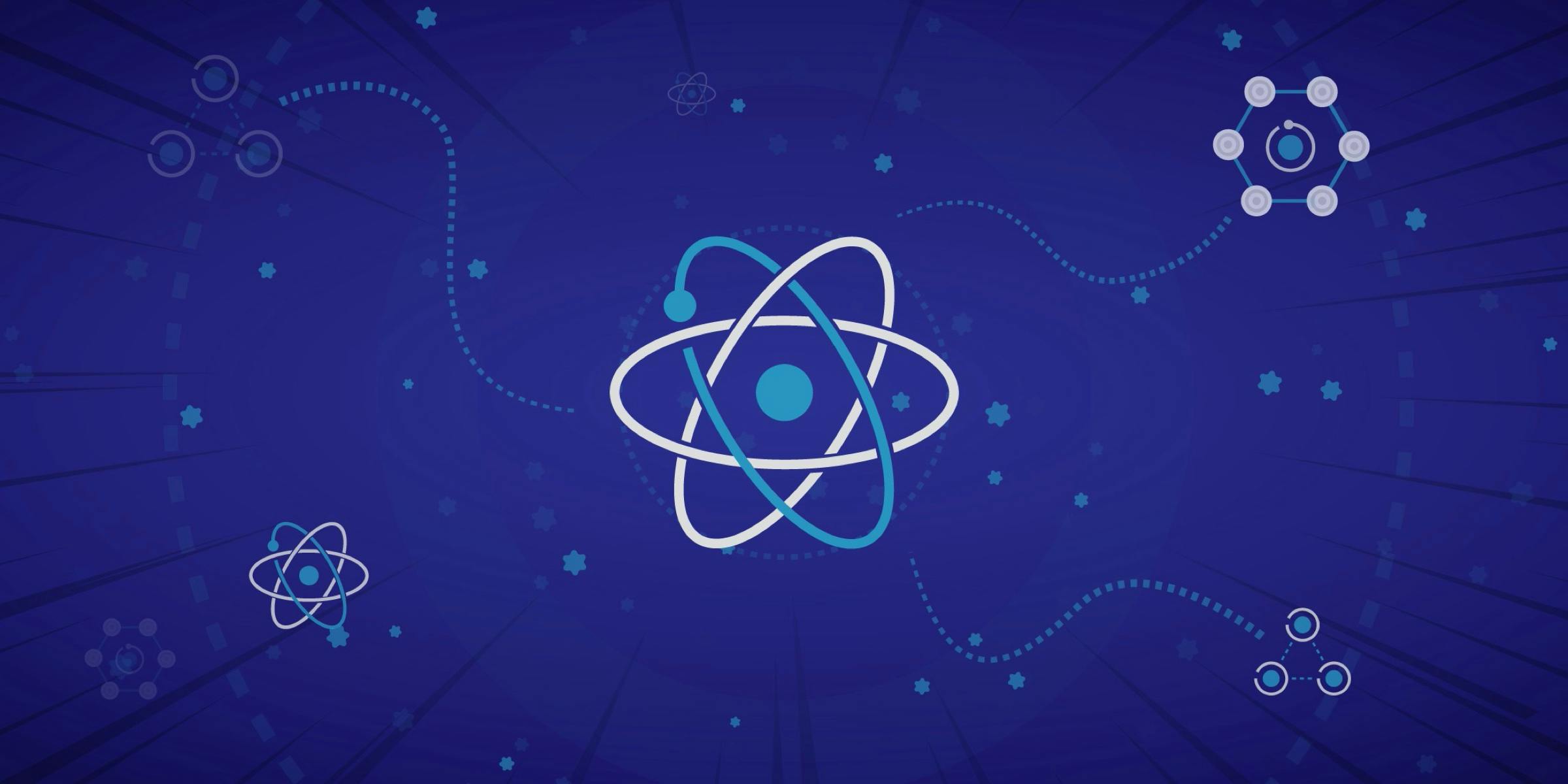
porGabriel Dürr M., São Paulo - SP
React JS is a popular JavaScript library that allows you to create interactive and dynamic user interfaces. It is used by thousands of developers worldwide to build scalable and efficient web applications. If you are new to React JS 18, this guide is for you.
Introduction to ReactJS
React JS is a JavaScript library for building user interfaces. It was developed by Facebook and maintained by an active community of developers. React JS is based on components, which are reusable building blocks that can be used to build user interfaces.
React JS is one of the most popular JavaScript libraries and is used by companies like Netflix, Airbnb, Instagram, Uber and many others. It is known for its efficiency and scalability, which makes it a popular choice for large-scale web applications.
Environment Setting
Before you start working with React JS, you need to set up the development environment. This involves installing Node.js and the React CLI. Node.js is a platform for running JavaScript outside the browser, while React CLI is a command-line tool for creating and managing React projects.
Creating a React project
NOTE: You need to install Node.js, go to the official website and download the latest version. To create a new React project, type the following command in the terminal:
npm create vite@latest NAME OF APP --template react
Components in React JS
Components are the foundation of building interfaces in React JS. They are like little building blocks that can be combined to create more complex interfaces. Each component has its own state and properties, which can be updated to reflect changes in the interface.
To create a component in React JS, you can use a function with the functional paradigm. The function receives the properties as arguments and returns what will be rendered in the interface.
type myComponentProps = {
title: string;
description: string;
};
export const MyComponent = ({ title, description }: myComponentProps) => {
return (
<div>
<h1>{title}</h1>
<p>{description}</p>
</div>
);
};
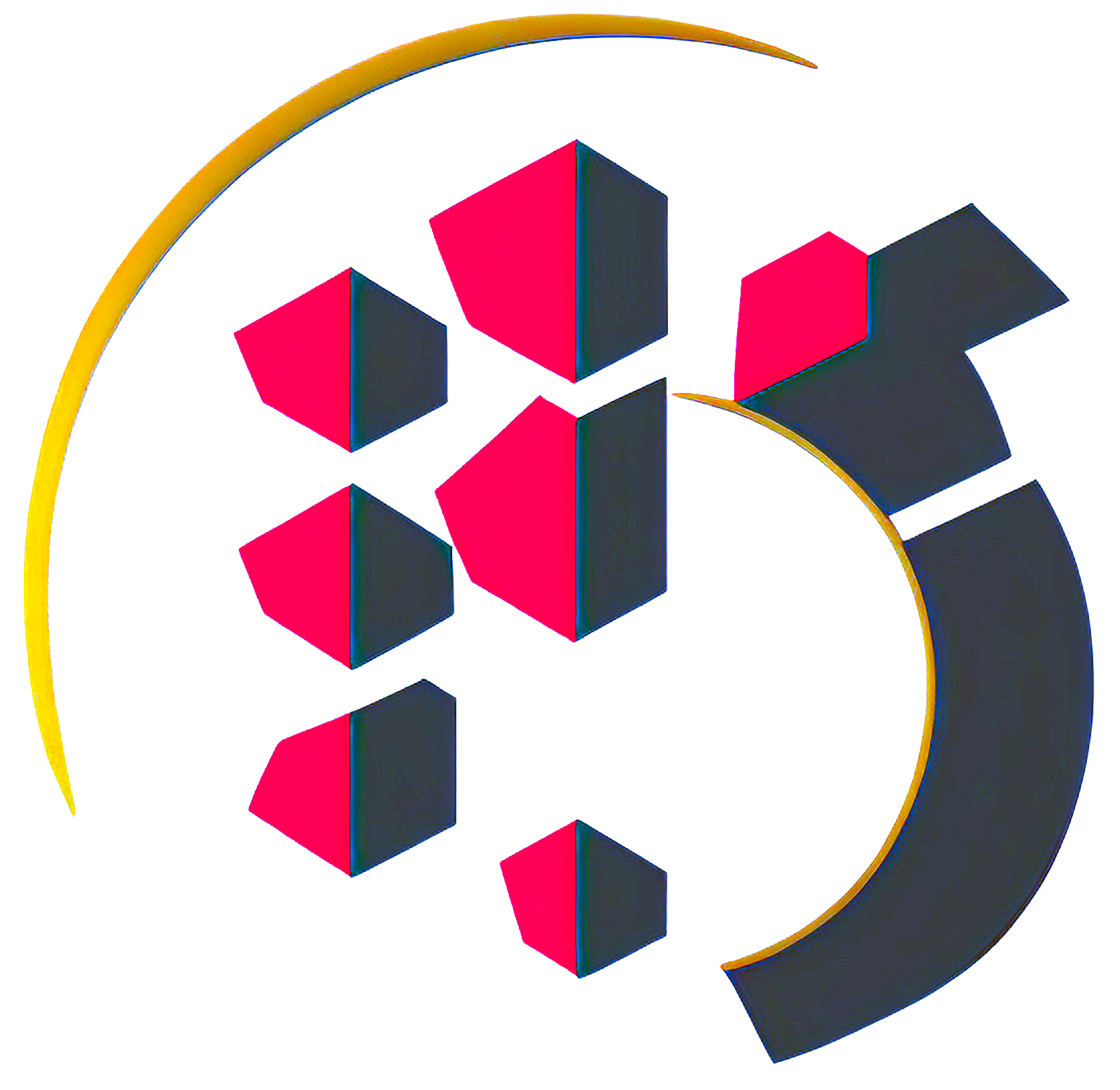
State in ReactJs
State management is an important part of app development in React JS. The state is an object that represents the current state of the interface. It can be updated to reflect changes to the interface.
React JS has a unique approach to state management. Instead of modifying the state directly, you should use the useState() method. useState() is used to update the state and notify React JS to re-render the interface.
import { useState } from "react";
type myComponentProps = {
title: string;
description: string;
};
export const MyComponent = ({ title, description }: myComponentProps) => {
const [count, setCount] = useState(0);
function handleClick() {
setCount(prevState => prevState + 1);
}
return (
<div>
<h1>{title}</h1>
<p>{description}</p>
<button onClick={handleClick}>Click Here</button>
</div>
);
};
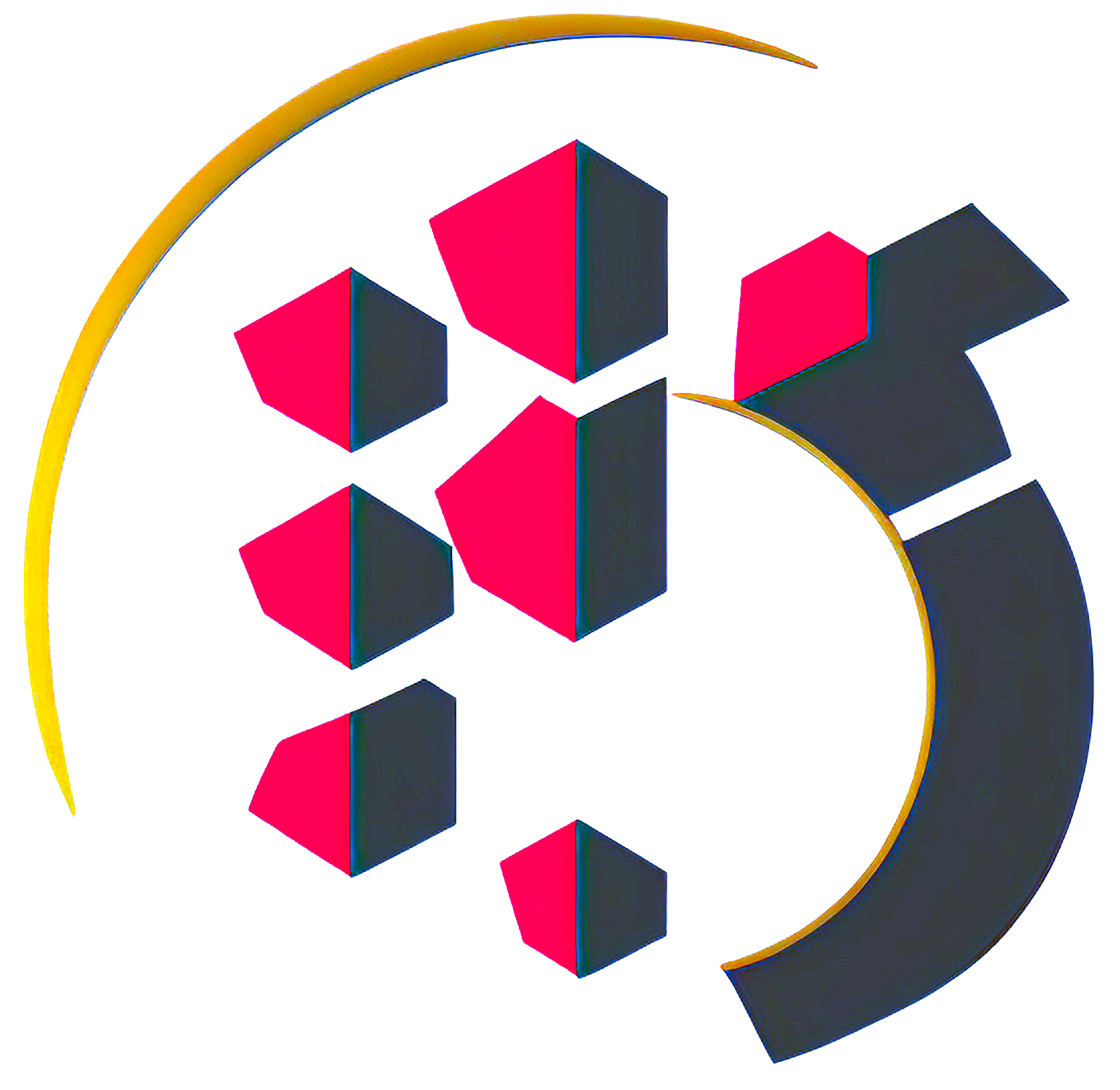
State management with Context API
State management is a fundamental aspect of ReactJS application development. The ContextAPI is a powerful tool that provides an efficient way to share state between components without the need to manually pass props.
To use the ContextAPI, you need to create a Context, which stores state and provides methods for updating it. Then you must wrap the components that need to access that state with the context provider. See the example:
import { createContext, useState } from 'react';
export const MyContext = createContext({});
const MyProvider = ({ children }) => {
const [myState, setMyState] = useState("gbdx");
return (
<MyContext.Provider value={{ myState, setMyState }}>
{children}
</MyContext.Provider>
);
};
export default MyProvider;
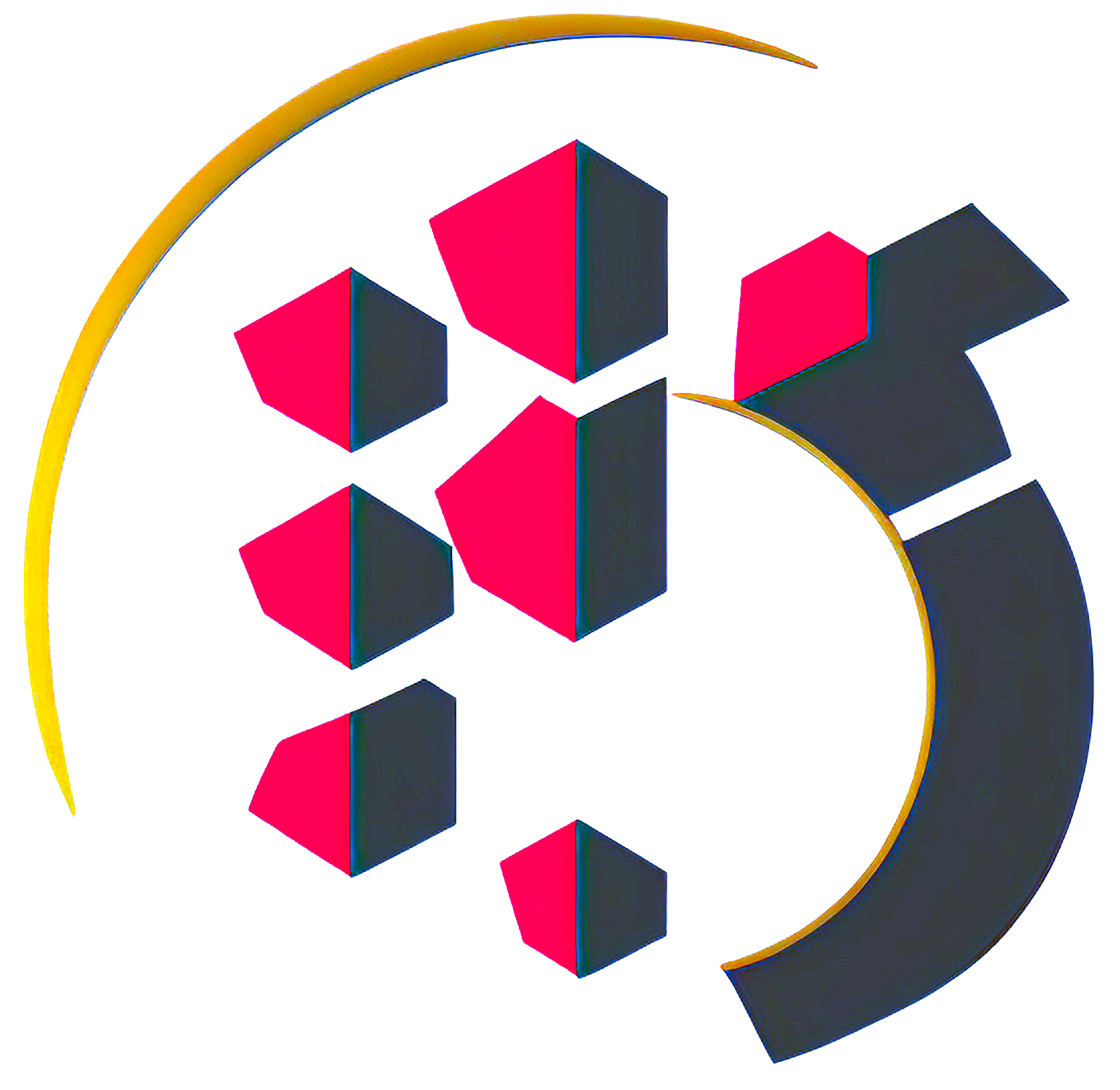
- This is a basic example of how to create a context with React's Context API. MyProvider is the component that will wrap the components that will have access to the context. It defines an initial state and makes it available through MyContext.Provider.
To use the context value in a component, just import MyContext and use useContext, as in the example below:
import { MyContext } from './MyProvider';
import React, { useContext } from 'react';
const MyComponent = () => {
const { myState, setMyState } = useContext(MyContext);
return (
<div>
<p>{myState}</p>
<button onClick={() => setMyState('new value')}>
CLick to Update
</button>
</div>
);
};
export default MyComponent;
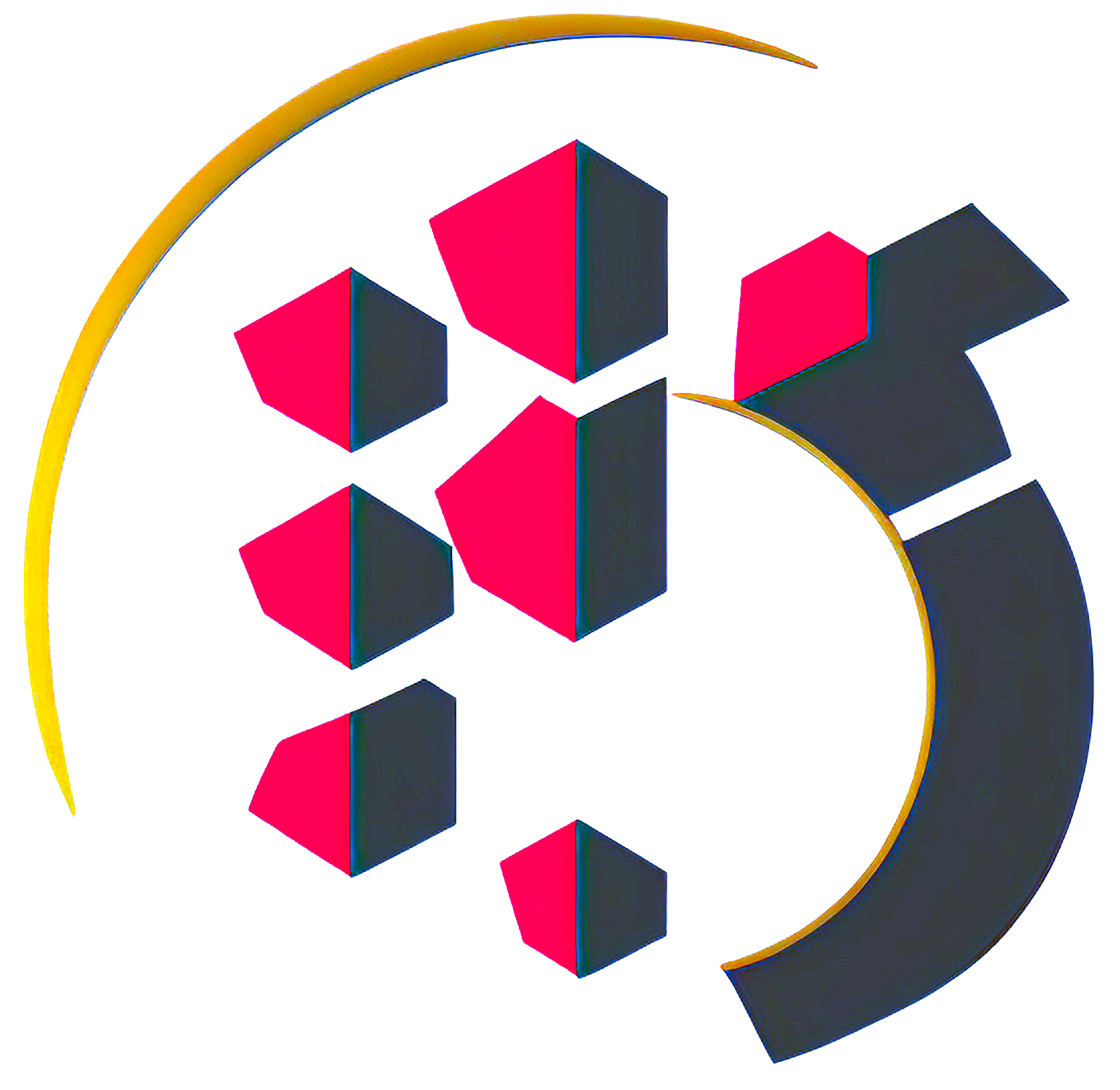
- By doing so, these components can access the state and update it as needed. Additionally, the ContextAPI allows us to use scope/need-based access to context properties in our application.
We can use the Context API provider in _app.js, that way the context will be provided to all components of your application that are rendered by it. This can be useful if you want to share the same context across multiple components or pages. For example, if you have a theme that you would like to apply throughout your application, you can define the Context API provider in _app.js to provide the theme for all components.
On the other hand, if you define the Context API provider on a specific component, it will only be provided for that component and its children. This is useful if you have a component that needs a specific context and is not relevant to the rest of the application.
import { MyProvider } from './hooks/MyProvider';
function MyApp({ Component, pageProps }) {
return (
<MyProvider>
<Component {...pageProps} />
</MyProvider>
);
}
export default MyApp;
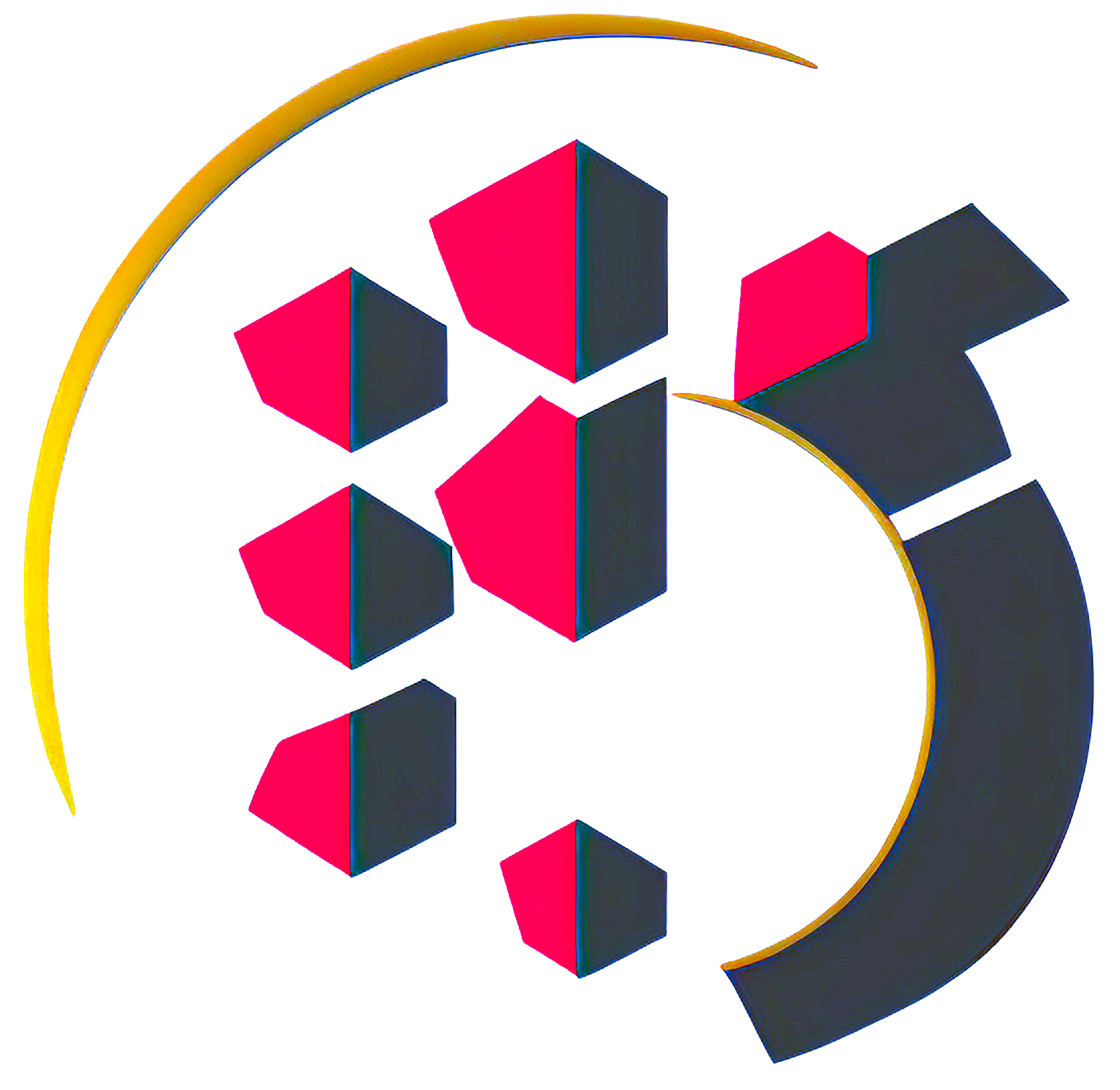
- However, it is important to remember that excessive use of the ContextAPI can make the application more complex and make the code difficult to maintain. Therefore, it is essential to carefully evaluate the need to use this tool in each case.
best practices
Here are some best practices for working with React JS:
- Separation of concerns
Separate code into smaller, more reusable components. Each component should have only one responsibility. This makes the code easier to understand and maintain.
- Dependency management
Use the npm package manager to manage your project's dependencies. This makes it easy to install and update your project's dependencies.
- Tests
Write tests to ensure your code is working correctly. Use tools like Jest and Enzyme to test React components. This helps ensure that your code is working correctly and helps reduce bugs and regression issues.
Conclusion
React JS is a powerful library for building user interfaces. With this guide, you should have a basic understanding to build awesome web apps. And if you want to go deeper, an amazing new official React JS documentation was created recently!